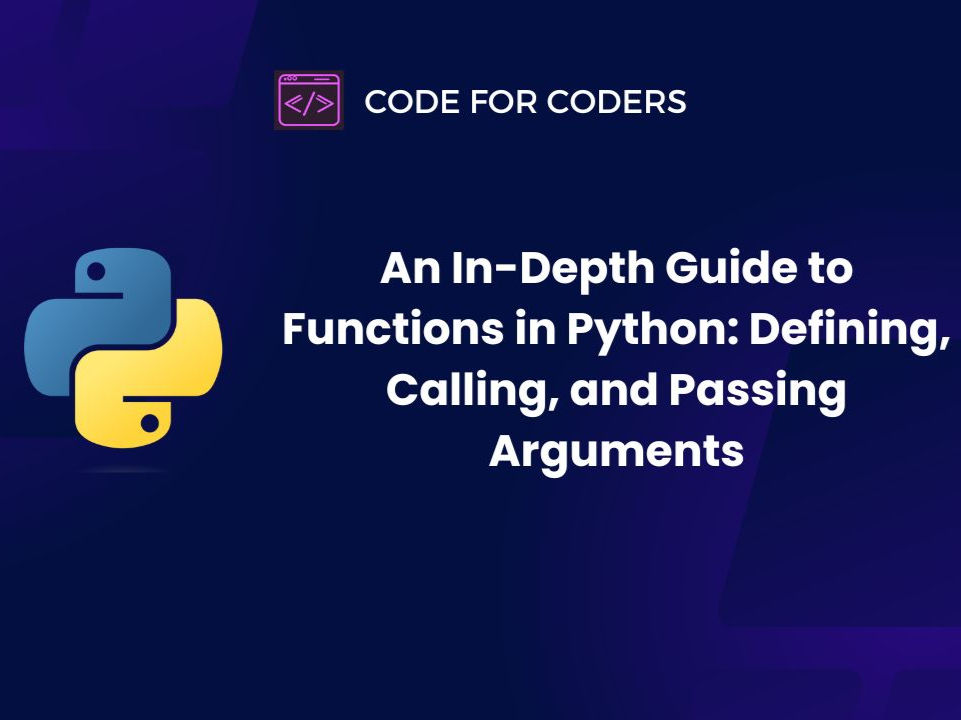
Introduction To Python Functions
Functions are essential building blocks of any programming language, enabling developers to organize code, improve reusability, and enhance maintainability. In Python, functions play a pivotal role, offering a flexible and expressive way to encapsulate logic and perform tasks. In this comprehensive guide, we will delve into the fundamentals of functions in Python, covering everything from defining and calling functions to passing arguments and returning values.
1. Defining Functions
In Python, functions are defined using the `def` keyword followed by the function name and a pair of parentheses. Optionally, parameters (also known as arguments) can be specified within the parentheses.
def greet():
print("Hello, world!")
# Calling the greet function
greet()
Output:
```
Hello, world!
```
In the example above, we define a simple function named `greet` that prints "Hello, world!" when called. Functions can contain any number of statements and can perform a wide range of tasks, from simple calculations to complex data processing.
2. Function Parameters
Functions in Python can accept parameters, allowing for the passing of data into the function for processing. Parameters are specified within the parentheses following the function name, and multiple parameters can be separated by commas.
def greet(name):
print("Hello, " + name + "!")
# Calling the greet function with a parameter
greet("Alice")
Output:
```
Hello, Alice!
```
In this example, the `greet` function accepts a single parameter `name`, which is then used within the function to customize the greeting message. When calling the function, we provide the argument `"Alice"`, which gets passed to the `greet` function and used to personalize the greeting.
3. Default Parameters
Python allows specifying default values for function parameters, enabling the definition of functions with optional arguments. Default parameters are specified by assigning a value to the parameter within the function definition.
def greet(name="world"):
print("Hello, " + name + "!")
# Calling the greet function without providing a parameter
greet()
# Calling the greet function with a parameter
greet("Bob")
```
Output:
```
Hello, world!
Hello, Bob!
In this example, the `greet` function has a default parameter `name` set to `"world"`. If no argument is provided when calling the function, it defaults to using the value `"world"`. However, callers can still override the default value by providing their own argument, as demonstrated in the second function call.
4. Returning Values
Functions in Python can return values using the `return` statement. The `return` statement allows functions to produce output that can be used by the caller for further processing.
def add(a, b):
return a + b
# Calling the add function and storing the result in a variable
result = add(3, 5)
print("The sum is:", result)
```
Output:
```
The sum is: 8
```
In this example, the `add` function takes two parameters `a` and `b` and returns their sum using the `return` statement. When calling the function with arguments `3` and `5`, the returned value `8` is stored in the `result` variable and subsequently printed to the console.
5. Calling Functions with Keyword Arguments
Python allows calling functions with keyword arguments, where arguments are specified by their parameter names. This feature enhances readability and allows for flexibility in function calls, especially when dealing with functions with multiple parameters.
def greet(first_name, last_name):
print("Hello, " + first_name + " " + last_name + "!")
# Calling the greet function with keyword arguments
greet(last_name="Doe", first_name="John")
```
Output:
```
Hello, John Doe!
```
In this example, the `greet` function is called with keyword arguments `first_name="John"` and `last_name="Doe"`. The order of the arguments does not matter since they are explicitly labeled with their parameter names.
6. Variable-Length Argument Lists
Python supports variable-length argument lists, allowing functions to accept an arbitrary number of arguments. This is achieved using special syntax with `*` for non-keyword variable-length arguments and `**` for keyword variable-length arguments.
def sum_values(*args):
total = 0
for num in args:
total += num
return total
# Calling the sum_values function with variable-length arguments
result = sum_values(1, 2, 3, 4, 5)
print("The sum is:", result)
```
Output:
```
The sum is: 15
```
In this example, the `sum_values` function accepts a variable-length argument list `args`, denoted by `*args`. The function then iterates over the provided arguments, summing them up and returning the total. When calling the function with arguments `1, 2, 3, 4, 5`, it calculates the sum `15`.
7. Passing Arguments by Reference vs. by Value
In Python, arguments are passed by reference for mutable objects (e.g., lists, dictionaries) and by value for immutable objects (e.g., integers, strings). Understanding this distinction is crucial for avoiding unexpected behavior when modifying arguments within functions.
def modify_list(lst):
lst.append(4)
my_list = [1, 2, 3]
modify_list(my_list)
print("Modified list:", my_list)
```
Output:
```
Modified list: [1, 2, 3, 4]
```
In this example, the `modify_list` function modifies the provided list `lst` by appending the value `4` to it. Since lists are mutable objects, changes made to the list within the function persist outside the function scope.
def modify_number(num):
num += 1
my_num = 10
modify_number(my_num)
print("Modified number:", my_num)
```
Output:
```
Modified number: 10
In contrast, when passing an immutable object like an integer to a function, modifications made to the argument within the function do not affect the original value outside the function scope. In this example, the `modify_number` function increments the provided number `num` by `1`, but the original value of `my_num` remains unchanged.
Conclusion
Functions are a cornerstone of Python programming, enabling developers to write modular, reusable, and maintainable code. By mastering the concepts of defining functions, passing arguments, and returning values, programmers can harness the full power of Python's functional capabilities.
In this guide, we've explored the fundamentals of functions in Python, covering topics such as defining functions, specifying parameters, returning values, and utilizing advanced features like default parameters and variable-length argument lists. Armed with this knowledge, developers can write cleaner, more efficient code and tackle a wide range of programming tasks with confidence.
Comentarios