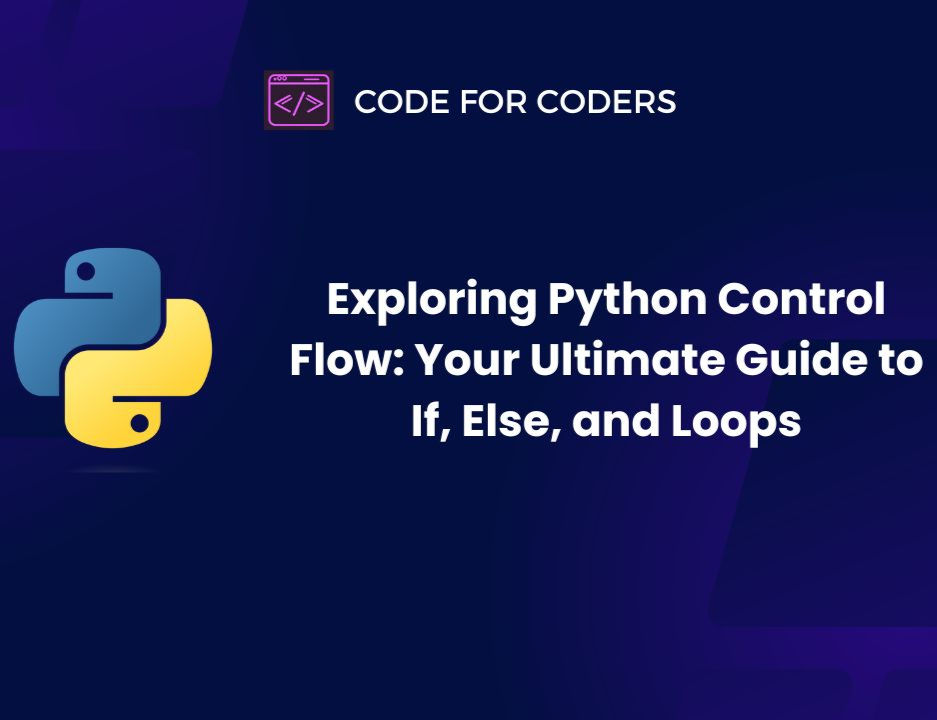
Python Control Flow Introduction :
Python, renowned for its simplicity and readability, offers powerful constructs for controlling the flow of execution within a program. Understanding these control flow mechanisms—such as if statements, else clauses, and various types of loops—is fundamental for writing efficient and expressive Python code. In this comprehensive guide, we'll delve into the intricacies of Python's control flow structures, accompanied by illustrative code examples.
1. If Statements
The cornerstone of conditional execution in Python is the `if` statement. It allows you to execute a block of code conditionally based on the evaluation of an expression.
x = 10
if x > 5:
print("x is greater than 5")
In this example, the code within the `if` block will only execute if the condition `x > 5` evaluates to `True`. If the condition is `False`, the block is skipped entirely.
1.1. Else Clauses
To complement `if` statements, Python provides the `else` clause, which allows you to specify a block of code to execute when the condition of the `if` statement evaluates to `False`.
x = 3
if x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
Here, if `x` is not greater than 5, the code within the `else` block will execute.
1.2. Elif Clauses
In scenarios where you have multiple conditions to evaluate, Python offers the `elif` clause, short for "else if". It allows you to chain multiple conditions together in a concise and readable manner.
x = 10
if x > 15:
print("x is greater than 15")
elif x > 5:
print("x is greater than 5 but not greater than 15")
else:
print("x is not greater than 5")
In this example, if `x` is greater than 15, the first block executes. If not, but `x` is greater than 5, the second block executes. Otherwise, the last block executes.
2. Loops
Loops are essential for iterating over sequences of data or performing repetitive tasks. Python provides several types of loops, including `for` loops, `while` loops, and nested loops.
2.1. For Loops
`for` loops are used to iterate over a sequence (such as a list, tuple, or string) or any iterable object.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
This loop iterates over each element in the `fruits` list and prints it.
2.2. Range Function
The `range()` function is commonly used in conjunction with `for` loops to generate a sequence of numbers.
for i in range(5):
print(i)
This loop prints the numbers from 0 to 4. The `range()` function can also accept start, stop, and step arguments to customize the sequence.
2.3. While Loops
`while` loops continue iterating as long as a specified condition is `True`.
i = 0
while i < 5:
print(i)
i += 1
This loop prints the numbers from 0 to 4, similar to the `for` loop example above.
3. Control Flow Keywords: Break and Continue
In addition to `if` statements and loops, Python provides two keywords—`break` and `continue`—to modify the flow of execution within loops.
3.1. Break
The `break` statement immediately terminates the innermost loop in which it appears.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
if fruit == "banana":
break
print(fruit)
This loop iterates over the `fruits` list and prints each fruit until it encounters "banana", at which point it exits the loop.
3.2. Continue
The `continue` statement skips the rest of the code inside a loop for the current iteration and proceeds to the next iteration.
for i in range(5):
if i == 2:
continue
print(i)
In this example, the loop prints all numbers from 0 to 4 except for 2, as the `continue` statement skips the `print(i)` statement when `i` equals 2.
4. Practical Examples
Let's explore a few practical examples that combine conditional statements and loops to solve real-world problems.
4.1. Finding Even Numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for num in numbers:
if num % 2 == 0:
print(num, "is even")
else:
print(num, "is odd")
This code iterates over the `numbers` list and prints whether each number is even or odd.
4.2. Calculating Factorials
n = 5
factorial = 1
for i in range(1, n + 1):
factorial *= i
print("Factorial of", n, ":", factorial)
This code calculates the factorial of a given number (`n`) using a `for` loop.
Conclusion
Understanding control flow constructs such as `if` statements, loops, and control flow keywords is essential for writing efficient and expressive Python code. By mastering these concepts and practicing their application in various scenarios, you can enhance your programming skills and tackle a wide range of problems effectively. Experiment with the provided examples and explore further to deepen your understanding of Python's control flow mechanisms.
Kommentare