Table of Contents:-
1. Introduction to Python
2. Setting Up Python Environment
3. Your First Python Program
4. Python Basics: Variables and Data Types
5. Python Control Flow: If, Else, and Loops
6. Functions in Python
7. Python Modules and Packages
8. File Handling in Python
9. Exception Handling in Python
10. Conclusion and Next Steps
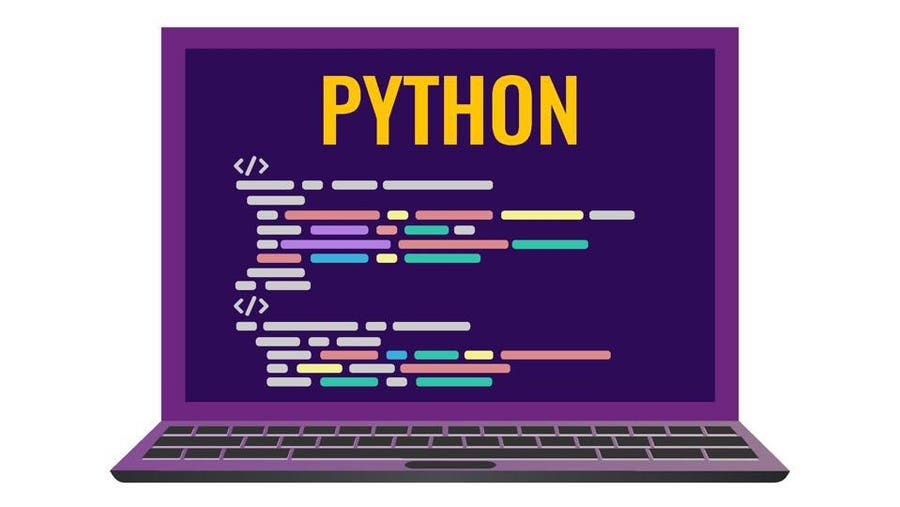
Python is a powerful and versatile programming language known for its simplicity and readability. Whether you're a complete beginner or an experienced programmer looking to learn a new language, Python is an excellent choice to start with. In this beginner's guide, we'll walk you through the fundamentals of Python programming and help you get started on your coding journey.
1. Introduction to Python
Python was created by Guido van Rossum and first released in 1991. It has since gained widespread popularity in various fields such as web development, data science, machine learning, and more. Python's syntax is clean and easy to understand, making it an ideal language for beginners.
2. Setting Up Python Environment
Before you can start coding in Python, you'll need to set up your development environment. Python is available for all major operating systems (Windows, macOS, and Linux) and can be easily installed from the official website (https://www.python.org/).
Follow the installation instructions for your operating system to install Python on your computer. Once installed, you can use the Python interpreter to run Python code from the command line or use an Integrated Development Environment (IDE) such as PyCharm, Visual Studio Code, or IDLE for a more user-friendly coding experience.
3. Your First Python Program
Now that you have Python installed, let's write our first Python program. Open your preferred text editor or IDE and create a new file called `hello.py`. In this file, type the following code:
print("Hello, world!")
Save the file and then open a terminal or command prompt. Navigate to the directory where you saved `hello.py` and run the following command:
python hello.py
You should see the output `Hello, world!` printed to the console. Congratulations! You've just written and executed your first Python program.
4. Python Basics: Variables and Data Types
In Python, variables are used to store data values. Variables can store different types of data such as numbers, strings, lists, tuples, dictionaries, and more. Python is dynamically typed, which means you don't need to specify the data type when declaring a variable.
# Example of variables and data types in Python
x = 10 # integer
y = 3.14 # float
name = "John Doe" # string
is_student = True # boolean
5. Python Control Flow: If, Else, and Loops
Python provides several control flow statements such as `if`, `else`, `elif`, and loops (`for` and `while`) to control the flow of execution in a program.
# Example of if-else statement in Python
x = 10
if x > 0:
print("Positive number")
elif x == 0:
print("Zero")
else:
print("Negative number")
6. Functions in Python
Functions are a block of reusable code that performs a specific task. In Python, you can define functions using the `def` keyword.
# Example of a function in Python
def greet(name):
print("Hello, " + name + "!")
# Call the function
greet("Alice")
**7. Python Modules and Packages**
Python modules are files containing Python code that can be imported and used in other Python programs. A package is a collection of Python modules.
# Example of importing modules in Python
import math
print(math.sqrt(16)) # Output: 4.0
8. File Handling in Python
Python provides built-in functions for file handling, allowing you to read from and write to files on your computer.
# Example of file handling in Python
with open("data.txt", "w") as file:
file.write("Hello, world!\n")
file.write("Python is awesome!")
**9. Exception Handling in Python**
Exception handling allows you to handle errors gracefully and prevent your program from crashing.
# Example of exception handling in Python
try:
x = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
10. Conclusion and Next Steps
Congratulations! You've completed the beginner's guide to Python programming.
Now you have a rough idea of how to learn Python programming. In the next articles, we'll delve deeper into Python Programming Concepts and understand them in depth level.
Comments