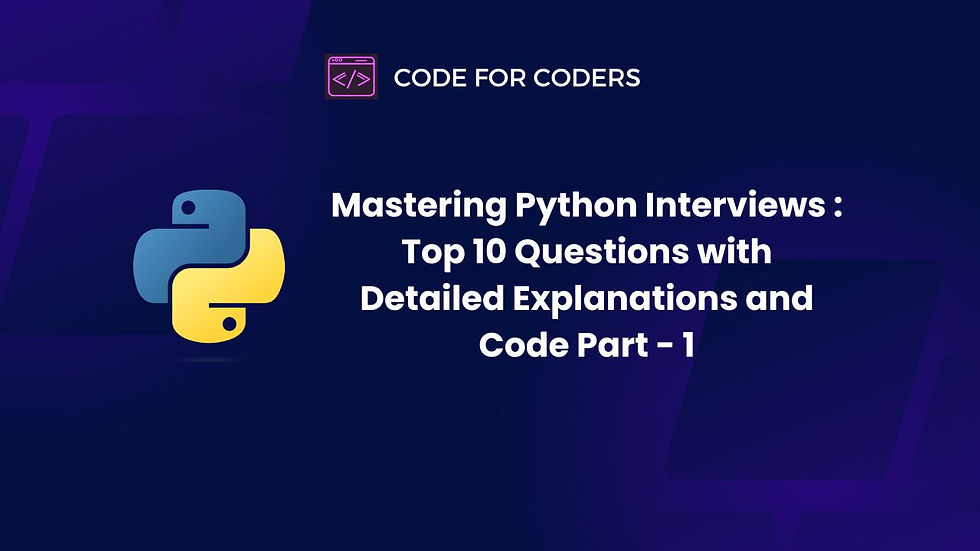
Python programming language has emerged as one of the most popular choices for developers due to its simplicity, versatility, and readability. Whether you're a seasoned Python developer or just starting your journey in programming, preparing for Python interviews is essential to land your dream job. To help you ace your next Python interview, we've compiled a list of the top 20 Python programming interview questions, complete with detailed explanations and code examples.
1. What is Python? Explain its key features.
Python is a high-level, interpreted programming language known for its simplicity and readability. Its key features include:
- Easy-to-learn syntax: Python's syntax resembles plain English, making it accessible to beginners.
- Interpreted nature: Python code is executed line by line, facilitating rapid development and debugging.
- Dynamic typing: Variables in Python are dynamically typed, meaning their type is determined at runtime.
- Rich standard library: Python comes with a vast collection of built-in modules and libraries for various tasks.
2. What are the differences between Python 2 and Python 3?
Python 2 and Python 3 are two major versions of Python with several differences:
- Print Statement: Python 2 uses a `print` statement, whereas Python 3 uses the `print()` function.
- Division: In Python 2, the division of integers results in an integer, whereas in Python 3, it results in float.
- Unicode: Python 2 treats strings as ASCII by default, while Python 3 uses Unicode.
- `xrange()`: Python 2 has `xrange()` for iterating over a range of numbers efficiently, which is replaced by `range()` in Python 3.
# Python 2
print "Hello, World!"
# Python 3
print("Hello, World!")
3. What is PEP 8?
PEP 8 is the official style guide for Python code, outlining best practices for writing clean, readable, and maintainable code. It covers aspects such as indentation, naming conventions, whitespace usage, and code layout.
4. Explain list comprehension in Python.
List comprehension is a concise way to create lists in Python by iterating over an iterable and applying an expression to each element. It follows the syntax `[expression for item in iterable if condition]`.
# Example: Squaring numbers from 1 to 5 using list comprehension
squared_numbers = [x**2 for x in range(1, 6)]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
5. What are decorators in Python?
Decorators are a powerful feature in Python that allow you to modify or extend the behavior of functions or methods without directly modifying their code. They are implemented using the `@decorator_name` syntax.
# Example: Simple decorator to print function name before execution
def my_decorator(func):
def wrapper(*args, **kwargs):
print(f"Executing {func.__name__}...")
return func(*args, **kwargs)
return wrapper
@my_decorator
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Executing greet... Hello, Alice!
6. What is the difference between `__str__` and `__repr__`?
`__str__` and `__repr__` are both special methods used to represent objects as strings, but they serve different purposes:
- `__str__`: Returns the informal string representation of an object, intended for end-users.
- `__repr__`: Returns the official string representation of an object, intended for developers for debugging and logging.
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return f"({self.x}, {self.y})"
def __repr__(self):
return f"Point({self.x}, {self.y})"
point = Point(3, 5)
print(str(point)) # Output: (3, 5)
print(repr(point)) # Output: Point(3, 5)
7. Explain the difference between `__getattr__` and `__getattribute__`.
`__getattr__` and `__getattribute__` are both special methods used to handle attribute access in Python, but they differ in when they are invoked:
- `__getattr__`: Invoked when an attribute that doesn't exist is accessed.
- `__getattribute__`: Invoked every time an attribute is accessed, regardless of whether it exists or not.
class MyClass:
def __getattr__(self, name):
return f"Attribute '{name}' not found!"
def __getattribute__(self, name):
print(f"Accessing attribute '{name}'...")
return object.__getattribute__(self, name)
obj = MyClass()
print(obj.x) # Output: Accessing attribute 'x'... Attribute 'x' not found!
8. What is the difference between `==` and `is` operators?
- `==` operator compares the values of two objects.
- `is` operator checks if two objects refer to the same memory location.
x = [1, 2, 3]
y = [1, 2, 3]
print(x == y) # Output: True
print(x is y) # Output: False
9. Explain the use of `*args` and `**kwargs` in Python function definitions.
`*args` and `**kwargs` are special syntax in Python used to pass a variable number of arguments to a function:
- `*args`: Collects positional arguments into a tuple.
- `**kwargs`: Collects keyword arguments into a dictionary.
def my_function(*args, **kwargs):
print("Positional arguments:", args)
print("Keyword arguments:", kwargs)
my_function(1, 2, 3, name='Alice', age=30)
# Output:
# Positional arguments: (1, 2, 3)
# Keyword arguments: {'name': 'Alice', 'age': 30}
10. What are generators in Python?
Generators are functions that produce a sequence of values using the `yield` keyword, allowing you to iterate over the values one at a time. They are memory-efficient and suitable for generating large sequences of values lazily.
def countdown(n):
while n > 0:
yield n
n -= 1
for num in countdown(5):
print(num) # Output: 5 4 3 2 1
These are just the first ten questions in our list of the top 20 Python programming interview questions. Stay tuned for the second part of this article, where we'll cover the remaining questions in detail. With thorough preparation and practice, you'll be well-equipped to tackle any Python interview with confidence and competence.
Comments