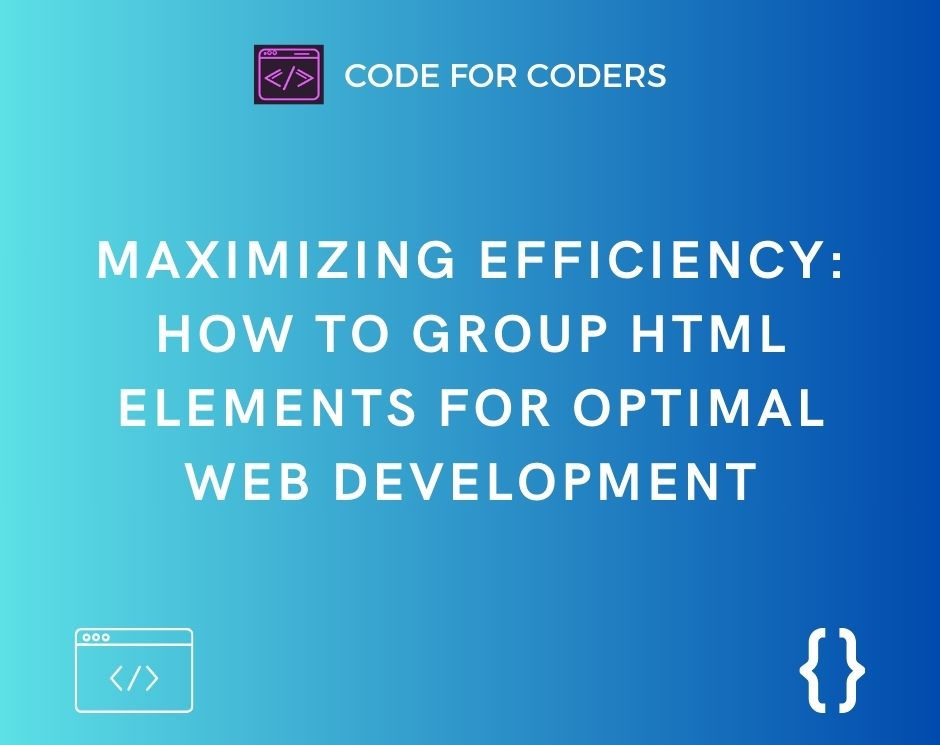
Introduction
HTML (HyperText Markup Language) is the backbone of the World Wide Web. It provides the structure and layout for web pages, enabling browsers to interpret and display content effectively. One of the fundamental aspects of HTML is the ability to group elements. Grouping elements allows web developers to organize content, apply styles, and manage page layouts efficiently. This article will delve into the importance of grouping elements, popular grouping tags such as `<div>`, and practical use cases for these tags in modern web development.
The Importance of Grouping HTML Elements
Grouping HTML elements serves several crucial purposes in web development:
1. Improved Readability and Maintainability: Grouping elements logically helps developers and future maintainers of the code understand the structure and flow of a webpage more easily. This is particularly important in complex websites with many nested elements.
2. Enhanced Styling and Layout Control: Grouping elements allows developers to apply CSS styles to entire sections of a webpage at once, ensuring a consistent look and feel. It also facilitates the use of CSS frameworks and grid systems for responsive design.
3. Better Accessibility: Properly grouped elements can improve the accessibility of a webpage. Screen readers and other assistive technologies can interpret the structure more accurately, providing a better experience for users with disabilities.
4. Efficient Scripting: JavaScript and other scripting languages can more easily manipulate grouped elements, making dynamic and interactive web pages more straightforward to implement.
Popular Grouping Tags in HTML
Several HTML tags are commonly used for grouping elements. These include `<div>`, `<span>`, `<section>`, `<article>`, `<nav>`, and more. Each tag has specific use cases and benefits. Let's explore some of these popular grouping tags in detail.
1. `<div>` (Division)
The `<div>` tag is one of the most versatile and widely used grouping tags in HTML. It is a block-level element that can contain other block-level and inline elements. The primary purpose of `<div>` is to group related content together.
Use Cases
- Creating Layouts: `<div>` tags are often used to create the overall structure of a webpage, including headers, footers, sidebars, and main content areas. For example:
<div class="header">Header Content</div>
<div class="sidebar">Sidebar Content</div>
<div class="main-content">Main Content</div>
<div class="footer">Footer Content</div>
- Styling Sections: Developers can apply CSS styles to `<div>` elements to style entire sections of a webpage. For instance:
.container {
width: 80%;
margin: 0 auto;
}
.header, .footer {
background-color: #333;
color: #fff;
}
- JavaScript Interactions: `<div>` elements can be easily targeted with JavaScript for dynamic behavior. For example:
<div id="content">This is some content.</div>
<button onclick="changeContent()">Change Content</button>
<script>
function changeContent() {
document.getElementById('content').innerText = 'Content has been
changed!';
}
</script>
2. `<span>`
The `<span>` tag is an inline-level element used to group inline elements for styling purposes. Unlike `<div>`, which is block-level, `<span>` does not affect the flow of the document.
Use Cases
- Styling Text: The `<span>` tag is often used to apply styles to a portion of text within a block of content. For example:
<p>This is a <span class="highlight">highlighted</span> word.</p>
- JavaScript Targeting: Similar to `<div>`, `<span>` can be targeted by JavaScript for dynamic updates. For example:
<p>This is a <span id="dynamicText">dynamic</span> word.</p>
<button onclick="updateText()">Update Text</button>
<script>
function updateText() {
document.getElementById('dynamicText').innerText = 'updated';
}
</script>
3. `<section>`
The `<section>` tag defines a section of a document, such as chapters, headers, footers, or any other thematic grouping of content.
Use Cases
- Thematic Grouping: The `<section>` tag is used to group related content that shares a common theme. For example:
<section>
<h2>Introduction</h2>
<p>This is the introduction section.</p>
</section>
<section>
<h2>Details</h2>
<p>This is the details section.</p>
</section>
- Semantic HTML: Using `<section>` improves the semantic meaning of the HTML document, making it more accessible and easier for search engines to index.
4. `<article>`
The `<article>` tag is intended to represent a self-contained piece of content that can be independently distributed or reused.
Use Cases
- Blog Posts: Each blog post on a website can be wrapped in an `<article>` tag. For example:
<article>
<h1>Blog Post Title</h1>
<p>This is the content of the blog post.</p>
</article>
- News Articles: News websites often use `<article>` tags for individual news stories.
5. `<nav>`
The `<nav>` tag defines a set of navigation links.
Use Cases
- Website Navigation: The `<nav>` tag is used to group navigation links, making it clear that these links are for navigating the site. For example:
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
Practical Applications
Building a Simple Webpage Layout
Let's build a simple webpage layout using some of the grouping tags we've discussed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Webpage Layout</title>
<style>
body {
font-family: Arial, sans-serif;
}
.container {
width: 80%;
margin: 0 auto;
}
.header, .footer {
background-color: #333;
color: #fff;
text-align: center;
padding: 10px 0;
}
.main {
display: flex;
}
.sidebar {
width: 25%;
background-color: #f4f4f4;
padding: 10px;
}
.content {
width: 75%;
padding: 10px;
}
</style>
</head>
<body>
<div class="container">
<div class="header">Header</div>
<div class="main">
<div class="sidebar">Sidebar</div>
<div class="content">
<section>
<h2>Section 1</h2>
<p>This is the first section of the main content.</p>
</section>
<section>
<h2>Section 2</h2>
<p>This is the second section of the main content.</p>
</section>
</div>
</div>
<div class="footer">Footer</div>
</div>
</body>
</html>
In this example, we use `<div>` for the main structural components, `<section>` to group thematic content, and CSS for styling.
Applying Dynamic Styles with JavaScript
Here's an example of how grouping tags can be manipulated with JavaScript to create interactive elements.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Elements</title>
<style>
.highlight {
background-color: yellow;
}
</style>
</head>
<body>
<div class="container">
<section>
<h2>Interactive Section</h2>
<p id="text">This text can be highlighted.</p>
<button onclick="highlightText()">Highlight Text</button>
</section>
</div>
<script>
function highlightText() {
document.getElementById('text').classList.toggle('highlight');
}
</script>
</body>
</html>
In this example, clicking the button toggles the `highlight` class on the paragraph, changing its background color.
Conclusion
Grouping HTML elements is an essential practice in web development that enhances the readability, maintainability, styling, accessibility, and interactivity of web pages. Tags like `<div>`, `<span>`, `<section>`, `<article>`, and `<nav>` provide powerful tools for organizing and structuring content. By understanding and effectively using these grouping tags, developers can create well-structured, semantic, and dynamic web pages that offer a better experience for users and maintainers alike. Whether building simple
Comments