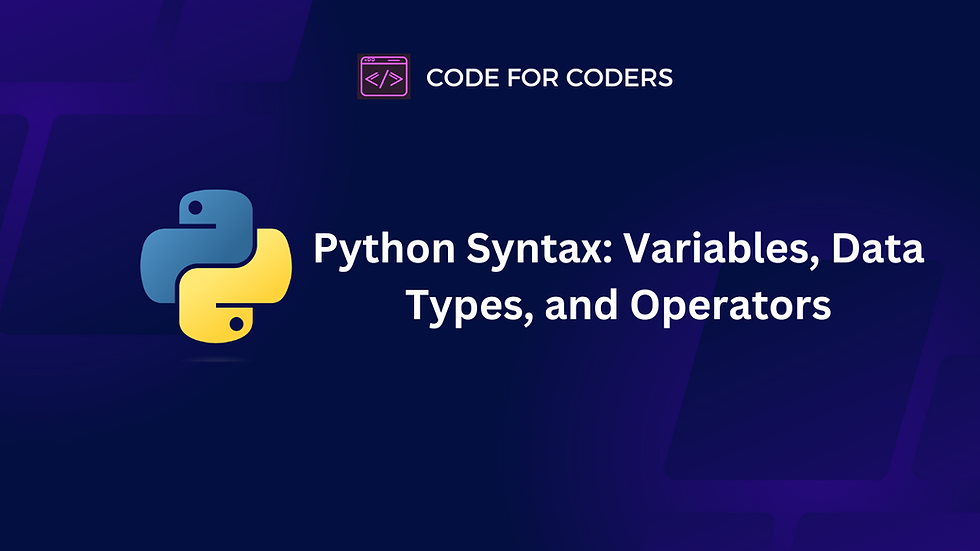
Python Syntax:
Python, renowned for its simplicity and readability, is a versatile programming language used across various domains, from web development to data analysis and machine learning. To embark on your Python journey, it's crucial to grasp the fundamental concepts of variables, data types, and operators. In this article, we'll delve into these core elements of Python syntax, accompanied by illustrative code examples.
Variables:
Variables serve as containers to store data values. In Python, you can create a variable and assign a value to it in a single line. Variable names can contain letters, numbers, and underscores but must start with a letter or underscore. Let's look at some examples:
# Variable assignment
x = 5
y = "Hello, Python!"
In the example above, `x` is assigned the integer value `5`, and `y` is assigned the string `"Hello, Python!"`. Python is dynamically typed, meaning you don't need to declare the variable type explicitly.
Data Types:
Python offers a rich set of data types, each tailored to handle specific kinds of data and operations. Let's delve deeper into each data type, exploring their characteristics, use cases, and common operations.
1. Integer:
Integers represent whole numbers without any fractional part. They can be positive, negative, or zero. In Python, integers are represented using the `int` type. You can perform arithmetic operations such as addition, subtraction, multiplication, and division on integers.
x = 10
y = -5
2. Float:
Floats, short for floating-point numbers, represent real numbers with a decimal point. They can also be expressed in scientific notation. Floats are represented using the `float` type in Python. Operations like addition, subtraction, multiplication, and division can be performed on floats.
pi = 3.14
height = 6.2
3. String:
Strings are sequences of characters enclosed within single, double, or triple quotes. They allow manipulation of textual data such as concatenation, slicing, and formatting. Strings are immutable, meaning their contents cannot be changed after creation.
name = "John"
message = 'Hello, World!'
multiline_string = """This is a multiline
string in Python."""
4. List:
Lists are ordered collections of items, which can be of different data types. They are mutable, allowing you to add, remove, or modify elements dynamically. Lists are defined using square brackets `[]`.
fruits = ["apple", "banana", "orange"]
numbers = [1, 2, 3, 4, 5]
mixed_list = [10, "Python", True]
5. Tuple:
Tuples are similar to lists but are immutable, meaning their contents cannot be modified after creation. They are defined using parentheses `()`. Tuples are often used to represent fixed collections of items.
coordinates = (10, 20)
rgb_color = (255, 0, 0) # Represents red color
6. Dictionary:
Dictionaries are unordered collections of key-value pairs. Each key is unique and maps to a corresponding value. Dictionaries are mutable and are defined using curly braces `{}`. They provide fast lookup for retrieving values based on keys.
person = {"name": "Alice", "age": 30, "city": "New York"}
student = {"id": 101, "name": "John", "grade": "A"}
7. Boolean:
Booleans represent truth values, `True` or `False`. They are primarily used for logical operations and conditional expressions. Booleans are essential for decision-making in programming.
is_python_fun = True
is_raining = False
Understanding these data types equips you with the necessary tools to handle diverse data and perform a wide range of operations in Python. As you gain proficiency, you'll leverage these data types effectively to build robust and efficient Python programs.
Operators:
Operators are symbols or special keywords used to perform operations on variables and values. Python supports various types of operators, including arithmetic, comparison, logical, assignment, and more. Let's examine some commonly used operators:
# Arithmetic operators
a = 10
b = 3
print(a + b) # Output:13 (Addition)
print(a - b) # Output:7 (Subtraction)
print(a * b) # Output:30 (Multiplication)
print(a / b) # Output:3.3333333333 (Division)
print(a % b) # Output:1 (Modulus)
print(a ** b) # Output:1000 (Exponentiation)
print(a // b) # Output: 3 (Floor division)
# Comparison operators
x = 5
y = 10
print(x == y) # Output: False (Equal to)
print(x != y) # Output: True(Not equal to)
print(x > y) # Output: False(Greater than)
print(x < y) # Output: True (Less than)
print(x >= y) # Output: False (Greater than or equal to)
print(x <= y) # Output: True (Less than or equal to)
# Logical operators
p = True
q = False
print(p and q) # Output: False (Logical AND)
print(p or q) # Output: True (Logical OR)
print(not p) # Output: False (Logical NOT)
# Assignment operators
z = 5
z += 3 # Equivalent to z = z + 3
z -= 2 # Equivalent to z = z - 2
z *= 4 # Equivalent to z = z * 4
z /= 2 # Equivalent to z = z / 2
z %= 3 # Equivalent to z = z % 3
Understanding operators is essential for performing various computations and making decisions in your Python programs.
Conclusion:
In this article, we've covered the foundational aspects of Python syntax, focusing on variables, data types, and operators. Mastering these concepts lays a solid groundwork for your Python programming journey. As you continue your exploration of Python, remember to practice writing code and experimenting with different syntax elements. With dedication and practice, you'll become proficient in Python programming and unlock its vast potential for building innovative applications. Happy coding!
Comments